Functions¶
Cognigy Functions are code blocks that can be executed within your Cognigy.AI installation and assist you with long-running asynchronous processes, such as interacting with third-party systems through HTTP API.
Functions are independent of Flows but can be triggered from a Flow and can also use the Inject & Notify APIs to send their results back into a Flow.
When running a Function, you can pass additional parameters, which are then available in the Function Code.
You can start using Functions by navigating to Build > Functions in the left-side menu.
Limitations¶
The maximum runtime for an instance of a Cognigy Function is limited to 15 minutes. If you have an On-premise environment, you can change this limit.
Configuration and Monitoring¶
The Function Editor lets you define a Code snippet in JavaScript or TypeScript language.
You can access the parameters
and api
objects from the Function's arguments.
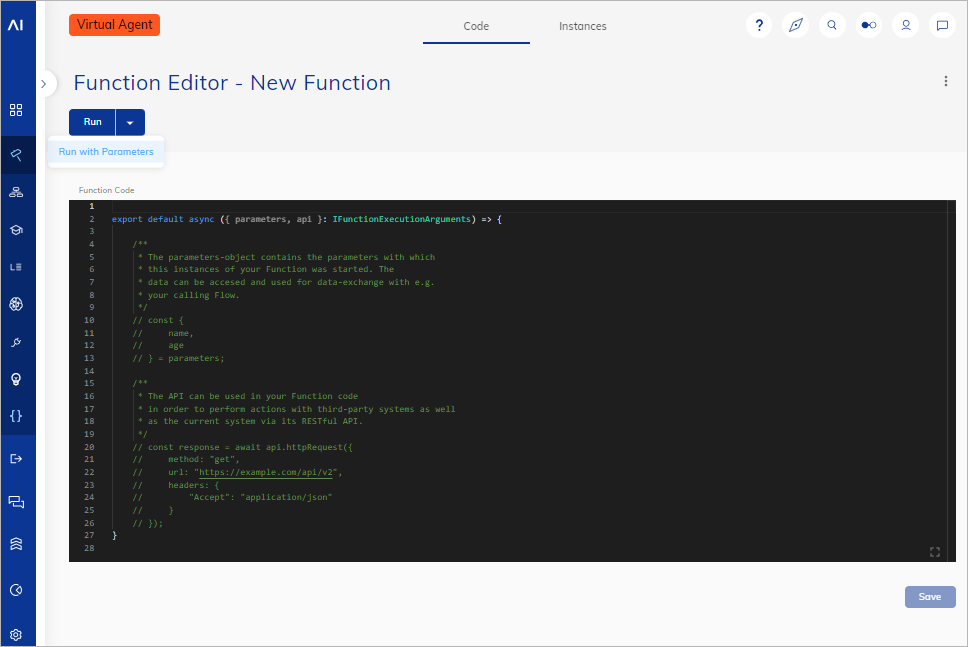
Interacting with Third-Party Systems¶
You can use HTTP requests to interact with third-party systems.
GET Request¶
const response = await api.httpRequest({
method: "get",
url: "https://example.com/api/v2",
headers: {
"Accept": "application/json"
}
});
POST Request¶
export default async ({ parameters, api }: IFunctionExecutionArguments) => {
/**
* The API can be used in your Function code
* in order to perform actions with third-party systems as well
* as the current system via its RESTful API.
*
* This is a POST request to the Cognigy API.
* For this demo, we use a function call to dynamically create a Flow.
*/
const response = await api.httpRequest({
method: "post",
url: "https://api-app.cognigy.ai/new/v2.0/flows?api_key=<your-api-key>",
headers: {
"Accept": "application/json"
},
data: {
"name": "A new flow",
"description": "A brief description",
"projectId": "<your-project-id>"
}
});
}
Interacting with Flows¶
You can use Functions to interact with Flows using the Inject & Notify APIs.
The following examples assume that you pass userId
and sessionId
through the Function's Parameters.
const { userId, sessionId } = parameters;
api.inject({
userId,
sessionId,
text: "This text was injected through a Function"
});
Performing a "Notify" call
const { userId, sessionId } = parameters;
api.notify({
userId,
sessionId,
text: "This text was injected through a Function"
});
Monitoring¶
By switching to the Instances tab, you can see an execution history for the currently edited Function. Each execution will list its current execution status, the trigger source, the start time and the finish time.
By hovering an item, you can also see the parameters that were provided for this individual execution.
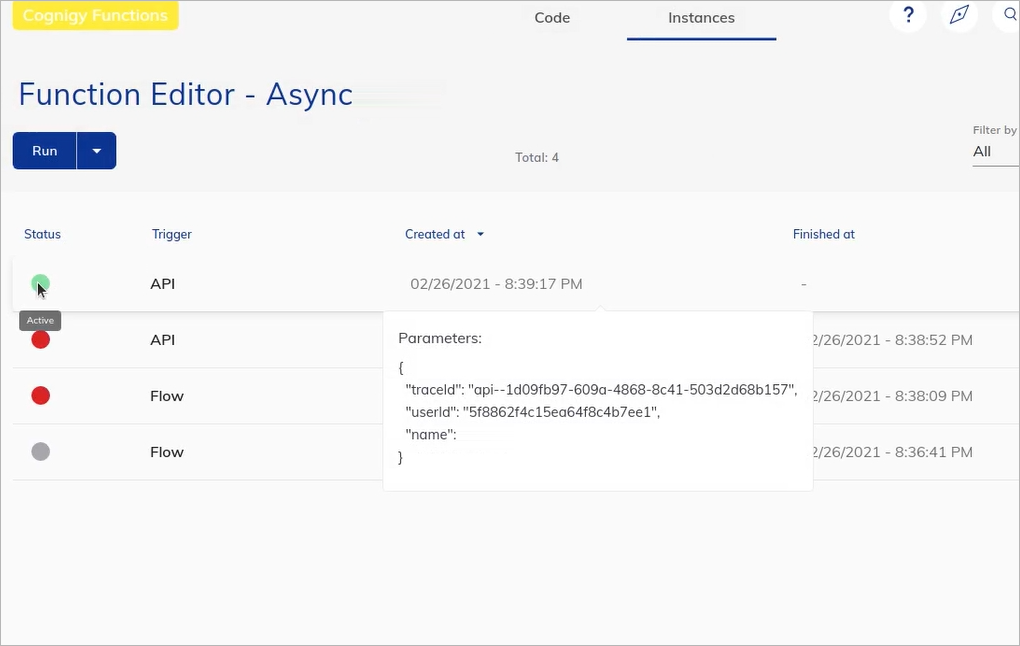
Triggering a Function¶
Cognigy Functions can be triggered in three different ways:
Using the UI¶
On the Function Editor page, click the Run button in the upper-left corner of the page. This action will run the Function without parameters.
To run a Function with parameters, click the Arrow button next to the Run button. This action will open a dialog, where you can define additional parameters as a JSON object.
Using the REST API¶
You can trigger a Function by using the REST API. For more information, read the OpenAPI documentation.
Using a Node¶
To trigger a Function from a Flow, use the Trigger Function Node.