Copilot: IFrame Tile¶
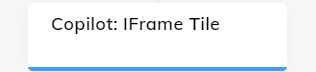
Description¶
This Node lets you embed external websites directly into the AI Copilot Workspace. To do that, specify a URL for the external content you want to display. You can present web pages, forms, or applications within the AI Copilot workspace without redirecting human agents to an external site.
Settings¶
Parameter | Type | Description |
---|---|---|
Tile ID | CognigyScript | The ID that you created in the AI Copilot configuration. |
IFrame URL | URL | The URL to render inside the Widget. |
JSON Data | JSON | The Data to send to the IFrame as a postMessage event. |
Passing JSON Data into the IFrame¶
When you use a Copilot: IFrame Tile Node, JSON data is passed into the IFrame code using the postMessage method. To receive and process the passed JSON in your widget, add an event listener to the Copilot HTML Tile Node and handle the data accordingly.
window.addEventListener("message", function (event) {
console.log("Content of message: " + event.data);
});
Example of an HTML page hosted on your website:
<!DOCTYPE html>
<html>
<head>
<title>Iframe Example</title>
</head>
<body>
<script>
let i = 0; // Handle postMessage from iframe parent
// Update the body document content every second
setInterval(function () {
i++;
document.body.innerHTML = '<h1>Iframe Tile ' + i + '</h1>';
}, 1000);
// Listen for messages from the parent window
window.addEventListener('message', function (event) {
// We only accept messages from ourselves
// if (event.source != window) return; // Uncomment this line if needed
console.log('Content of message: ' + event.data);
});
</script>
<h1>Iframe Tile</h1>
</body>
</html>
Sending JSON Data back to the Flow¶
You can send data back to the Flow by using the Postback feature.
The Postback feature allows you to send data either back to the Flow or chat, or directly to the widget interface. This feature can be useful for updating the state of your widget based on human agent interactions. These interactions can include actions such as submitting forms, updating display elements, or notifying the system of changes made by the human agent.
The Postback feature can be configured in the following ways:
Using SDK.postback¶
Follow these steps to implement postback for your widget:
-
Add the
SDK.postback
function to the HTML page. Make sure this code is included after the SDK script is loaded:<button type="button" onclick="SDK.postback('value');">Yes</button>
<button type="button" onclick="SDK.postback({ "key": "value" });">Yes</button>
-
Click the button in the widget in the AI Copilot workspace. Triggering this action will send an injected message formatted as follows:
{ "data": { "_cognigy": { "_agentAssist": { "type": "submit", "payload": "value" } } } }
To allow human agents to view this object, add {{ input.data._cognigy._agentAssist }}
to the Text field in the Say Node of your Copilot Flow.
This Node should be placed below the Node with the SDK.postback
function.
The output will be available in the chat message and visible only to human agents.
Example¶
In this example, the SDK.postback
function sends the value of name
back to the user.
If name
is falsy, meaning the user did not enter a name, the else
block is executed, and the function calls setMessage('Please enter your name.');
.
This message will appear in the widget, prompting the user to provide their name and reminding them of the required input.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0"/>
<title>Minimal React Widget</title>
<!-- External React libraries -->
<script src="https://unpkg.com/react@18/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@18/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
</head>
<body>
<div id="root"></div>
<script type="text/babel">
const { useState } = React;
// This event listener processes incoming data updates
window.addEventListener("message", function (event) {
console.log("Content of message: " + event.data);
});
function ReactWidget() {
const [name, setName] = useState('');
const [message, setMessage] = useState('');
const handleData = () => {
if (name) {
setMessage('Your name is ' + name);
SDK.postback(name);
} else {
setMessage('Please enter your name.');
}
};
return (
<div>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
placeholder="Name"
/>
<button onClick={handleData}>Submit</button>
<p>{message}</p>
</div>
);
}
ReactDOM.render(<ReactWidget />, document.getElementById('root'));
</script>
<button type="button" onclick="SDK.postback('yes');">yes</button>
</body>
</html>
Using the Copilot: Send Data¶
In the AI Copilot Flow, below the Copilot: IFrame Tile Node, add a Copilot: Send Data. In the JSON field of the Copilot: Send Data Node, specify the parameters you want to pass as metadata to the Copilot: IFrame Tile Node to update the content.